HTMLヘルパーのRadioButtonを使ったプログラム
今回は、HTMLヘルパーのラジオボタン(RadioButton)を使ったサンプルプログラムを作りましょう。
ラジオボタンは、以下のようなHTMLの要素で、複数の項目から1つだけ選択することができます。
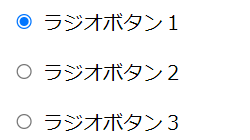
新規プロジェクトの作成
新規にプロジェクトを作成します。
今回のサンプルプログラムは、プロジェクト名を「HelperRadioButton」として作成します。
プロジェクトの作成方法は、こちらの記事を参照してください。
ビューモデルの作成
ビューモデルを作成します。
選択されたラジオボタンを保持するためのプロパティを、String型で定義します。
今回は、1つのグループのラジオボタンを作成するため、プロパティは1つだけ定義します。
1 2 3 |
Public Class ViewModel Public Property RadioButton As String End Class |
コントローラーの作成
今回は、コントローラーを1つだけ作成します。
この中に、入力用のビューを呼び出すInput()メソッドと、結果表示用のビューを呼び出すResult()メソッドを作成します。
Result()メソッドには、引数として選択されたラジオボタンの値を格納したモデルが渡されます。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
Imports System.Web.Mvc Namespace Controllers Public Class HomeController Inherits Controller Function Input() As ActionResult Return View() End Function Function Result(vm As ViewModel) As ActionResult Return View(vm) End Function End Class End Namespace |
入力画面用ビューの作成
入力画面用のビューを作成します。
HTMLヘルパーのHtml.RadioButtonFor()メソッドを使って、ラジオボタンを作成します。
Html.RadioButtonFor()メソッドの使い方は、以下の通りです。
@Html.RadioButtonFor(Function(model) model.プロパティ名, 値)
「Function(model) model.プロパティ」の部分は、ラムダ式といわれるもので、名前のない関数です。
Functionにmodelが渡され、modelの中のプロパティをHtml.RadioButtonFor()メソッドに引数として渡しています。
modelの内容は、コントローラーから渡されます。
上記では、Functionの引数をmodelとしていますが、名前はなんでも構いません。
詳しくは、以下のマイクロソフトの公式サイトを参照してください。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
@ModelType ViewModel @Code ViewData("Title") = "Input" End Code @Using Html.BeginForm("Result", "Home", FormMethod.Post) @<p> @Html.RadioButtonFor(Function(model) model.RadioButton, "1", New With {.id = "Radio1"}) @Html.Label("ラジオボタン1", New With {.for = "Radio1"}) </p> @<p> @Html.RadioButtonFor(Function(model) model.RadioButton, "2", New With {.id = "Radio2"}) @Html.Label("ラジオボタン2", New With {.for = "Radio2"}) </p> @<p> @Html.RadioButtonFor(Function(model) model.RadioButton, "3", New With {.id = "Radio3"}) @Html.Label("ラジオボタン3", New With {.for = "Radio3"}) </p> @<p><input type="submit" value="送信"></p> End Using |
RadioButtonFor()メソッドでは、プロパティ名がそのままidとnameになってしまうので、IDを個別に設定するために、New With {.id="ID名"}を指定します。
また、Html.Label()メソッドで、New With {.for="ID名"}を指定することで、ラベル部分をクリックしても選択きるようになります。
結果表示用ビューの作成
どのラジオボタンがチェックされたかどうかを表示するためのビューを作成します。
コントローラーから渡されたビューモデルのプロパティの値を参照して、該当のメッセージを表示します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
@ModelType ViewModel @Code ViewData("Title") = "Result" End Code <h3>ラジオボタン</h3> <p> @Code Select Case Model.RadioButton Case "1" @<p>ラジオボタン1を選択</p> Case "2" @<p>ラジオボタン2を選択</p> Case "3" @<p>ラジオボタン3を選択</p> Case Else @<p>選択なし</p> End Select End Code </p> <a href="/Home/Input/">戻る</a> |
表示レイアウトの編集
ビューを作成すると、自動的に「Views/Shared/_Layout.vbhtml」が作成されるので、これを以下のように編集します。
1 2 3 4 5 6 7 8 9 10 11 |
<!DOCTYPE html> <html lang="ja"> <head> <meta charset="utf-8" /> <title>@ViewBag.Title - My ASP.NET Application</title> <link href="~/Content/Site.css" rel="stylesheet" type="text/css" /> </head> <body> @RenderBody() </body> </html> |
プログラムの実行
ラジオボタンが3つ用意されているので、いづれかを選択して、「送信」ボタンを押下します。
また、ラベル部分を押下しても選択されます。
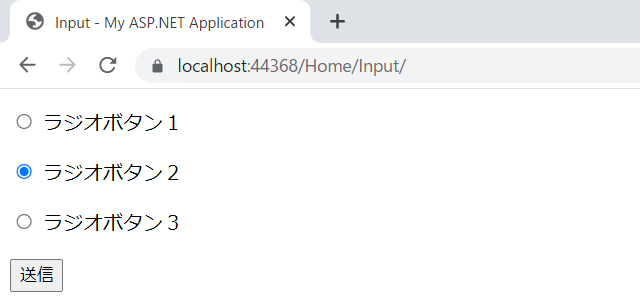
選択したラジオボタンの値をSelect Case文で分岐させ、該当するメッセージを表示します。
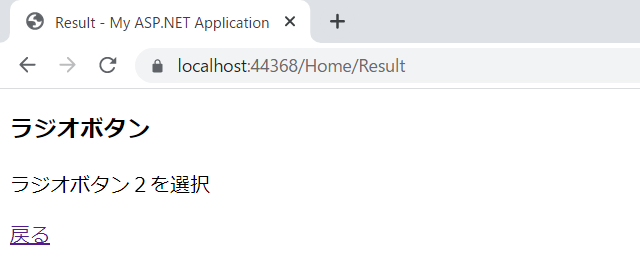