HTMLヘルパーのTextBoxを使ったプログラム
今回は、HTMLヘルパーのテキストボックス(TextBox)を使ったサンプルプログラムを作りましょう。
テキストボックスは、以下のようなHTMLの要素で、値を入力させるボックスです。
入力内容を数字のみや日付形式といった制限を付けることができます。
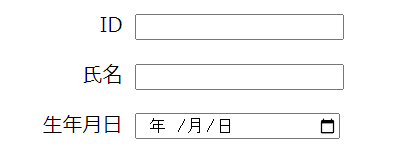
新規プロジェクトの作成
新規にプロジェクトを作成します。
今回のサンプルプログラムは、プロジェクト名を「HelperTextBox」として作成します。
プロジェクトの作成方法は、こちらの記事を参照してください。
ビューモデルの作成
ビューモデルを作成します。
数字のみ、文字列、日付形式の値を保持するためのプロパティを、それぞれ定義します。
ID(Id)は4桁のゼロ詰め、生年月日(Birthday)は「yyyy年MM月dd日」形式にします。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
Imports System.ComponentModel Imports System.ComponentModel.DataAnnotations Public Class ViewModel <DisplayName("ID")> <DisplayFormat(DataFormatString:="{0:0000}")> Public Property Id As Integer <DisplayName("氏名")> Public Property Name As String <DisplayName("生年月日")> <DisplayFormat(DataFormatString:="{0:yyyy年MM月dd日}")> Public Property Birthday As Date End Class |
コントローラーの作成
今回は、コントローラーを1つだけ作成します。
この中に、入力用のビューを呼び出すInput()メソッドと、結果表示用のビューを呼び出すResult()メソッドを作成します。
Result()メソッドには、引数としてテキストボックスに入力された値を格納したモデルが渡されます。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
Imports System.Web.Mvc Namespace Controllers Public Class HomeController Inherits Controller Function Input() As ActionResult Return View() End Function Function Result(vm As ViewModel) As ActionResult Return View(vm) End Function End Class End Namespace |
入力画面用ビューの作成
入力画面用のビューを作成します。
HTMLヘルパーのHtml.TextBoxFor()メソッドを使って、テキストボックスを作成します。
Html.TextBoxFor()メソッドの使い方は、以下の通りです。
@Html.TextBoxFor(Function(model) model.プロパティ名 , 属性)
「Function(model) model.プロパティ」の部分は、ラムダ式といわれるもので、名前のない関数です。
Functionにmodelが渡され、modelの中のプロパティをHtml.TextBoxFor()メソッドに引数として渡しています。
modelの内容は、コントローラーから渡されます。
上記では、Functionの引数をmodelとしていますが、名前はなんでも構いません。
詳しくは、以下のマイクロソフトの公式サイトを参照してください。
また、オプションで属性を指定することができます。
属性は、type、size、maxlengthなどが指定でき、「New With {.type="number"}」のように記述します。
属性を複数指定したい場合は、「New With {.type="number", size="20"」のようにカンマ(,)で区切ります。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
@ModelType ViewModel @Code ViewData("Title") = "Input" End Code @Using Html.BeginForm("Result", "Home", FormMethod.Post) @<div> <div class="label"> <p class="label">@Html.LabelFor(Function(model) model.Id)</p> <p class="label">@Html.LabelFor(Function(model) model.Name)</p> <p class="label">@Html.LabelFor(Function(model) model.Birthday)</p> </div> <div class="input"> <p>@Html.TextBoxFor(Function(model) model.Id, New With {.type = "number", .max = "9999", .class = "frame"})</p> <p>@Html.TextBoxFor(Function(model) model.Name, New With {.maxlength = "10", .class = "frame"})</p> <p>@Html.TextBoxFor(Function(model) model.Birthday, New With {.type = "date", .class = "frame"})</p> <p><input type="submit" value="送信" /></p> </div> </div> End Using |
結果表示用ビューの作成
テキストボックスに入力した値を表示するためのビューを作成します。
入力した値を、Html.DisplayFor()メソッドを使って表示します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
@ModelType ViewModel @Code ViewData("Title") = "Result" End Code <h3>テキストボックス</h3> <div class="container"> <div class="label"> <p class="label">@Html.LabelFor(Function(model) model.Id)</p> <p class="label">@Html.LabelFor(Function(model) model.Name)</p> <p class="label">@Html.LabelFor(Function(model) model.Birthday)</p> </div> <div class="input"> <p>@Html.DisplayFor(Function(model) model.Id)</p> <p>@Html.DisplayFor(Function(model) model.Name)</p> <p>@Html.DisplayFor(Function(model) model.Birthday)</p> </div> </div> <a href="/Home/Input/">戻る</a> |
表示レイアウトの編集
ビューを作成すると、自動的に「Views/Shared/_Layout.vbhtml」が作成されるので、これを以下のように編集します。
1 2 3 4 5 6 7 8 9 10 11 |
<!DOCTYPE html> <html lang="ja"> <head> <meta charset="utf-8" /> <title>@ViewBag.Title - My ASP.NET Application</title> <link href="~/Content/Site.css" rel="stylesheet" type="text/css" /> </head> <body> @RenderBody() </body> </html> |
スタイルシートの修正
このプロジェクトで使用するスタイルシート(Site.css)を、以下のように修正します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
div.container { overflow: hidden; } div.label { width: 100px; float: left; text-align: right; } div.input { width: 200px; float: left; } p.label { padding: 0 10px 0 0; } .frame { width: 160px; } |
プログラムの実行
テキストボックスに値を入力して、「送信」ボタンを押下します。
それぞれの入力内容が、ビューモデルで指定されたフォーマットで表示されます。
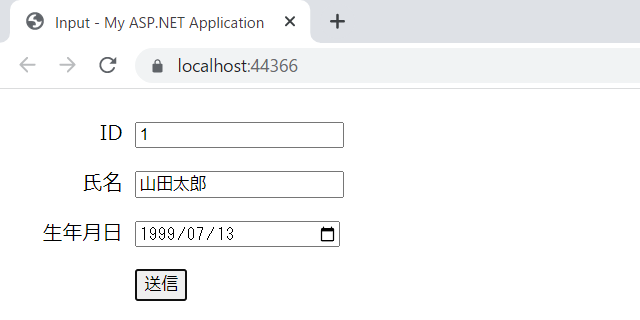
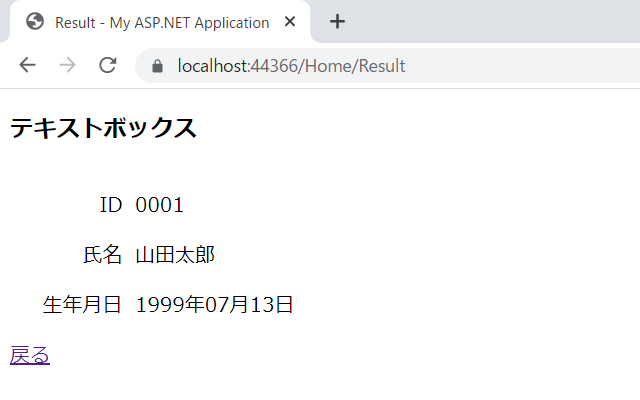