データベースのデータを更新するプログラム
今回は、こちらの記事で作成したプログラムに、データの更新処理を追加します。
データ一覧画面に更新用のリンクを追加
更新画面を呼び出すリンクを、一覧画面に追加します。
呼び出すコントローラーはUpdateController、呼び出すメソッドはUpdate()とします。
また、HTMLヘルパーのActionLink()メソッドは、コントローラーとNew Withでオプションを指定しているため、最後にNothingが必要になります。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
@ModelType IEnumerable(Of Emp) @Code ViewData("Title") = "Index" End Code <table> <tr> <th>@Html.ActionLink(Html.DisplayNameFor(Function(model) model.Id).ToString, "Sort", New With {.skey = "Id"})</th> <th>@Html.ActionLink(Html.DisplayNameFor(Function(model) model.Name).ToString, "Sort", New With {.skey = "Name"})</th> <th>@Html.ActionLink(Html.DisplayNameFor(Function(model) model.Address).ToString, "Sort", New With {.skey = "Address"})</th> <th>@Html.ActionLink(Html.DisplayNameFor(Function(model) model.Birthday).ToString, "Sort", New With {.skey = "Birthday"})</th> <th>@Html.ActionLink(Html.DisplayNameFor(Function(model) model.Email).ToString, "Sort", New With {.skey = "Email"})</th> <th></th> </tr> @For Each item In Model @<tr> <td>@Html.DisplayFor(Function(model) item.Id)</td> <td>@Html.DisplayFor(Function(model) item.Name)</td> <td>@Html.DisplayFor(Function(model) item.Address)</td> <td>@Html.DisplayFor(Function(model) item.Birthday)</td> <td>@Html.DisplayFor(Function(model) item.Email)</td> <td>@Html.ActionLink("更新", "Update", "Update", New With {.id = item.Id}, Nothing)</td> </tr> Next </table> <div class="index-link"> @Html.ActionLink("新規登録", "Insert", "Insert") </div> |
下図のように、更新画面へのリンクがデータ一覧の横に配置されます。
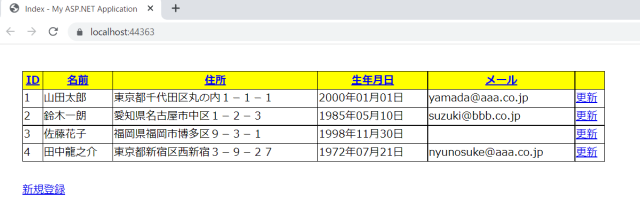
データ更新用のコントローラーを作成
新規にデータ更新用のコントローラーを作成します。
コントローラーには、データ入力用(Update)、データ確認用(UpdateConfirm)、データ更新用(UpdateDb)の3つのメソッドを作成します。
Update()メソッドは、選択したデータのIDが渡され、そのIDをキーとして、Find()メソッドを使ってEmpテーブルからデータを取得します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
Imports System.Web.Mvc Namespace Controllers Public Class UpdateController Inherits Controller Private db As New TestDbEntities Function Update(id As Integer) As ActionResult Dim emp As Emp = db.Emp.Find(id) Return View(emp) End Function <HttpPost> Function UpdateConfirm(emp As Emp) As ActionResult Return View("UpdateConfirm", emp) End Function <HttpPost> Function UpdateDb(emp As Emp) As ActionResult Dim model As New DbModel model.UpdateEmp(emp) Return RedirectToAction("Index", "Home") End Function End Class End Namespace |
データ更新用の処理を追加
既存のDbModelクラスに、データベースにデータを更新するメソッド(UpdateEmp)を追加します。
引数で渡されたEmpモデルの状態をModifiedを使って更新済みとした後に、SaveChanges()メソッドで、データベースの状態を保存します。
また、37行目のEntityState.Modifiedを使うために、System.Data.Entityをインポートする必要があります。
データ更新に関する詳しい内容は、以下のマイクロソフトの公式サイトを参照してください。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
Imports System.Data.Entity Imports System.Web.Mvc Public Class DbModel Function GetEmp(skey As String) As List(Of Emp) Dim emp As List(Of Emp) Using db As New TestDbEntities Select Case skey Case "Id" emp = db.Emp.OrderBy(Function(s) s.Id).ToList Case "Name" emp = db.Emp.OrderBy(Function(s) s.Name).ToList Case "Address" emp = db.Emp.OrderBy(Function(s) s.Address).ToList Case "Birthday" emp = db.Emp.OrderBy(Function(s) s.Birthday).ToList Case "Email" emp = db.Emp.OrderBy(Function(s) s.Email).ToList Case Else emp = db.Emp.OrderBy(Function(s) s.Id).ToList End Select End Using Return emp End Function Sub InsertEmp(emp As Emp) Using db As New TestDbEntities db.Emp.Add(emp) db.SaveChanges() End Using End Sub Sub UpdateEmp(emp As Emp) Using db As New TestDbEntities db.Entry(emp).State = EntityState.Modified db.SaveChanges() End Using End Sub End Class |
データ更新用のビューを作成
データの値を更新するビューを作成します。
Formの送信先に、UpdateコントローラーのUpdateConfirm()メソッドを指定します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
@ModelType Emp @Code ViewData("Title") = "Update" End Code <div class="input-container"> <div class="input-box1"> <p class="input-label">@Html.LabelFor(Function(model) model.Id):</p> <p class="input-label">@Html.LabelFor(Function(model) model.Name):</p> <p class="input-label">@Html.LabelFor(Function(model) model.Address):</p> <p class="input-label">@Html.LabelFor(Function(model) model.Birthday):</p> <p class="input-label">@Html.LabelFor(Function(model) model.Email):</p> </div> <div class="input-box2"> @Using (Html.BeginForm("UpdateConfirm", "Update", FormMethod.Post)) @Html.HiddenFor(Function(model) model.Id) @<p>@Html.DisplayFor(Function(model) model.Id)</p> @<p>@Html.TextBoxFor(Function(model) model.Name, New With {.maxlength = 20})</p> @<p>@Html.TextBoxFor(Function(model) model.Address, New With {.maxlength = 200, .size = 50})</p> @<p>@Html.TextBoxFor(Function(model) model.Birthday, "{0:yyyy-MM-dd}", New With {.type = "date"})</p> @<p>@Html.TextBoxFor(Function(model) model.Email, New With {.maxlength = 30})</p> @<div class="button"> <input type="submit" value="更新" /> <input type="button" value="戻る" onclick="backIndex()" /> </div> End Using </div> </div> |
「戻る」ボタンは、登録処理と同様、JavaScriptを使って、HomeコントローラーのIndex()メソッドに飛ぶようにしています。
データ更新画面は、以下のようになります。
元のデータが、そのままボックスに表示されます。
なお、IDはプライマリーキーなので、変更できないようにしています。
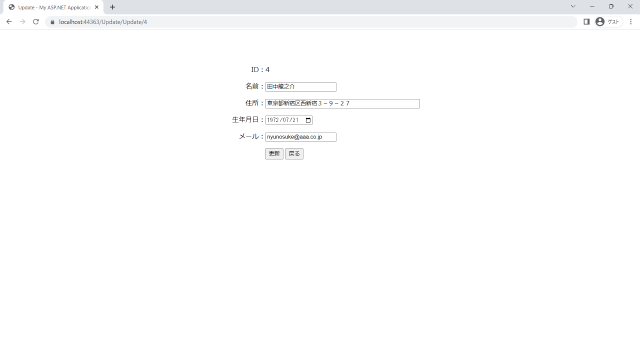
データ確認用のビューを作成
更新したデータを確認するためのビューを作成します。
値を表示するDisplayFor()メソッドは、Formでデータを渡すことができないので、HiddenFor()メソッドを使って、値をUpdateDb()メソッドに渡します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
@ModelType Emp @Code ViewData("Title") = "UpdateConfirm" End Code <div class="input-container"> <div class="input-box1"> <p class="input-label">@Html.LabelFor(Function(model) model.Id):</p> <p class="input-label">@Html.LabelFor(Function(model) model.Name):</p> <p class="input-label">@Html.LabelFor(Function(model) model.Address):</p> <p class="input-label">@Html.LabelFor(Function(model) model.Birthday):</p> <p class="input-label">@Html.LabelFor(Function(model) model.Email):</p> </div> <div class="input-box2"> @Using (Html.BeginForm("UpdateDb", "Update", FormMethod.Post)) @Html.HiddenFor(Function(model) model.Id) @Html.HiddenFor(Function(model) model.Name) @Html.HiddenFor(Function(model) model.Address) @Html.HiddenFor(Function(model) model.Birthday) @Html.HiddenFor(Function(model) model.Email) @<p>@Html.DisplayFor(Function(model) model.Id)</p> @<p>@Html.DisplayFor(Function(model) model.Name)</p> @<p>@Html.DisplayFor(Function(model) model.Address)</p> @<p>@Html.DisplayFor(Function(model) model.Birthday)</p> @<p>@Html.DisplayFor(Function(model) model.Email)</p> @<div class="button"> <input type="submit" value="更新" /> <input type="button" value="戻る" onclick="backIndex()" /> </div> End Using </div> </div> |
データ確認画面は、以下のようになります。
以下の例では、住所を変更しています。
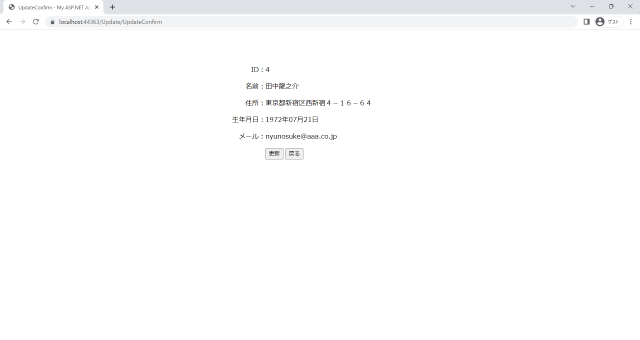
「更新」ボタンを押下すると、更新したデータがデータベースに登録され、一覧画面に戻ります。
一覧画面では、更新したデータが表示されます。
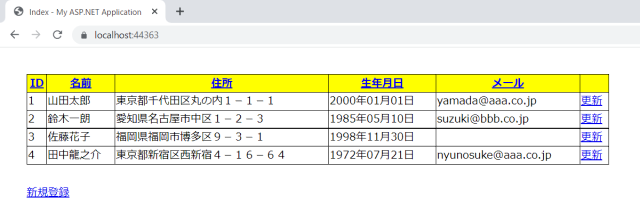