データベースにデータを登録するプログラム
今回は、こちらの記事で作成したプログラムを元に、データベースにデータを登録する処理を追加します。
データ一覧画面に新規登録処理用のリンクを追加
新規登録処理を呼び出すリンクを、一覧画面に追加します。
呼び出すコントローラーはInsertController、呼び出すメソッドはInsert()とします。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
@ModelType IEnumerable(Of Emp) @Code ViewData("Title") = "Index" End Code <table> <tr> <th>@Html.ActionLink(Html.DisplayNameFor(Function(model) model.Id).ToString, "Sort", New With {.skey = "Id"})</th> <th>@Html.ActionLink(Html.DisplayNameFor(Function(model) model.Name).ToString, "Sort", New With {.skey = "Name"})</th> <th>@Html.ActionLink(Html.DisplayNameFor(Function(model) model.Address).ToString, "Sort", New With {.skey = "Address"})</th> <th>@Html.ActionLink(Html.DisplayNameFor(Function(model) model.Birthday).ToString, "Sort", New With {.skey = "Birthday"})</th> <th>@Html.ActionLink(Html.DisplayNameFor(Function(model) model.Email).ToString, "Sort", New With {.skey = "Email"})</th> </tr> @For Each item In Model @<tr> <td>@Html.DisplayFor(Function(model) item.Id)</td> <td>@Html.DisplayFor(Function(model) item.Name)</td> <td>@Html.DisplayFor(Function(model) item.Address)</td> <td>@Html.DisplayFor(Function(model) item.Birthday)</td> <td>@Html.DisplayFor(Function(model) item.Email)</td> </tr> Next </table> <div class="index-link"> @Html.ActionLink("新規登録", "Insert", "Insert") </div> |
下図のように、登録画面へのリンクがデータ一覧の下に配置されます。
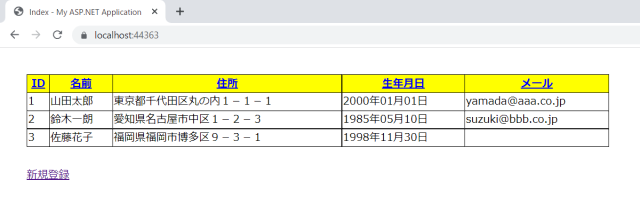
データ登録用のコントローラーを作成
新規にデータ登録用のコントローラーを作成します。
コントローラーには、データ入力用(Insert)、データ確認用(InsertConfirm)、データ登録用(InsertDb)の3つのメソッドを作成します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
Imports System.Web.Mvc Namespace Controllers Public Class InsertController Inherits Controller Function Insert() As ActionResult Return View() End Function <HttpPost> Function InsertConfirm(emp As Emp) As ActionResult Return View("InsertConfirm", emp) End Function <HttpPost> Function InsertDb(emp As Emp) As ActionResult Dim model As New DbModel model.InsertEmp(emp) Return RedirectToAction("Index", "Home") End Function End Class End Namespace |
データ登録用の処理を追加
既存のDbModelクラスに、データベースにデータを登録するメソッド(InsertEmp)を追加します。
引数で渡されたEmpモデルをAdd()メソッドで登録します。
データを登録した後に、SaveChanges()メソッドでデータベースの状態を保存します。
データ登録に関する詳しい内容は、以下のマイクロソフトの公式サイトを参照してください。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
Imports System.Web.Mvc Public Class DbModel Function GetEmp(skey As String) As List(Of Emp) Dim emp As List(Of Emp) Using db As New TestDbEntities Select Case skey Case "Id" emp = db.Emp.OrderBy(Function(s) s.Id).ToList Case "Name" emp = db.Emp.OrderBy(Function(s) s.Name).ToList Case "Address" emp = db.Emp.OrderBy(Function(s) s.Address).ToList Case "Birthday" emp = db.Emp.OrderBy(Function(s) s.Birthday).ToList Case "Email" emp = db.Emp.OrderBy(Function(s) s.Email).ToList Case Else emp = db.Emp.OrderBy(Function(s) s.Id).ToList End Select End Using Return emp End Function Sub InsertEmp(emp As Emp) Using db As New TestDbEntities db.Emp.Add(emp) db.SaveChanges() End Using End Sub End Class |
データ登録用のビューを作成
データの値を入力するビューを作成します。
Formの送信先に、InsertコントローラーのInsertConfirm()メソッドを指定します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
@ModelType Emp @Code ViewData("Title") = "Insert" End Code <div class="input-container"> <div class="input-box1"> <p class="input-label">@Html.LabelFor(Function(model) model.Id):</p> <p class="input-label">@Html.LabelFor(Function(model) model.Name):</p> <p class="input-label">@Html.LabelFor(Function(model) model.Address):</p> <p class="input-label">@Html.LabelFor(Function(model) model.Birthday):</p> <p class="input-label">@Html.LabelFor(Function(model) model.Email):</p> </div> <div class="input-box2"> @Using (Html.BeginForm("InsertConfirm", "Insert", FormMethod.Post)) @<p>@Html.TextBoxFor(Function(model) model.Id, New With {.maxlength = 10})</p> @<p>@Html.TextBoxFor(Function(model) model.Name, New With {.maxlength = 20})</p> @<p>@Html.TextBoxFor(Function(model) model.Address, New With {.maxlength = 200, .size = 50})</p> @<p>@Html.TextBoxFor(Function(model) model.Birthday, New With {.type = "date"})</p> @<p>@Html.TextBoxFor(Function(model) model.Email, New With {.maxlength = 30})</p> @<div class="button"> <input type="submit" value="確認" /> <input type="button" value="戻る" onclick="backIndex()"/> </div> End Using </div> </div> |
「戻る」ボタンは、JavaScriptを使って、HomeコントローラーのIndex()メソッドに飛ぶようにしています。
1 2 3 |
function backIndex() { location.href = '/Home/Index'; } |
JavaScriptを使うにあたって、_Layout.vbhtmlにJavaScriptのリンクを貼っておきます。
1 2 3 4 5 6 7 8 9 10 11 12 |
<!DOCTYPE html> <html lang="ja"> <head> <meta charset="utf-8" /> <title>@ViewBag.Title - My ASP.NET Application</title> <link href="~/Content/Site.css" rel="stylesheet" type="text/css" /> <script src="~/Scripts/JavaScript.js"></script> </head> <body> @RenderBody() </body> </html> |
データ登録画面は、以下のようになります。
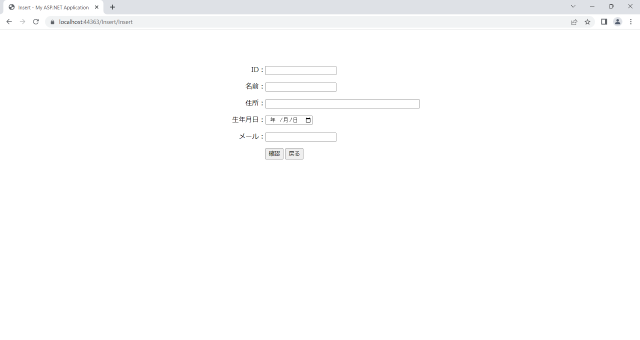
データ確認用のビューを作成
入力したデータを確認するためのビューを作成します。
値を表示するDisplayFor()メソッドは、Formでデータを渡すことができないので、HiddenFor()メソッドを使って、値をInsertDb()メソッドに渡します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
@ModelType Emp @Code ViewData("Title") = "InsertConfirm" End Code <div class="input-container"> <div class="input-box1"> <p class="input-label">@Html.LabelFor(Function(model) model.Id):</p> <p class="input-label">@Html.LabelFor(Function(model) model.Name):</p> <p class="input-label">@Html.LabelFor(Function(model) model.Address):</p> <p class="input-label">@Html.LabelFor(Function(model) model.Birthday):</p> <p class="input-label">@Html.LabelFor(Function(model) model.Email):</p> </div> <div class="input-box2"> @Using (Html.BeginForm("InsertDb", "Insert", FormMethod.Post)) @Html.HiddenFor(Function(model) model.Id) @Html.HiddenFor(Function(model) model.Name) @Html.HiddenFor(Function(model) model.Address) @Html.HiddenFor(Function(model) model.Birthday) @Html.HiddenFor(Function(model) model.Email) @<p>@Html.DisplayFor(Function(model) model.Id)</p> @<p>@Html.DisplayFor(Function(model) model.Name)</p> @<p>@Html.DisplayFor(Function(model) model.Address)</p> @<p>@Html.DisplayFor(Function(model) model.Birthday)</p> @<p>@Html.DisplayFor(Function(model) model.Email)</p> @<div class="button"> <input type="submit" value="登録" /> <input type="button" value="戻る" onclick="backIndex()" /> </div> End Using </div> </div> |
データ確認画面は、以下のようになります。
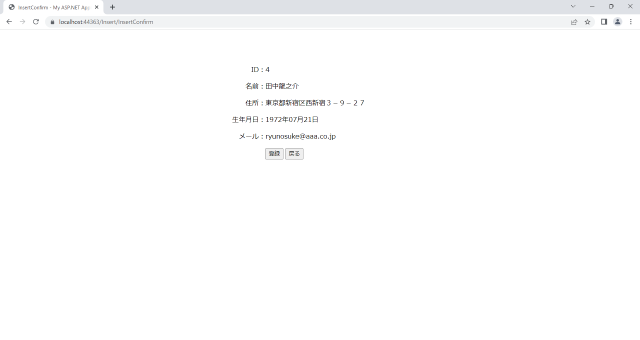
「登録」ボタンを押下すると、入力したデータがデータベースに登録され、一覧画面に戻ります。
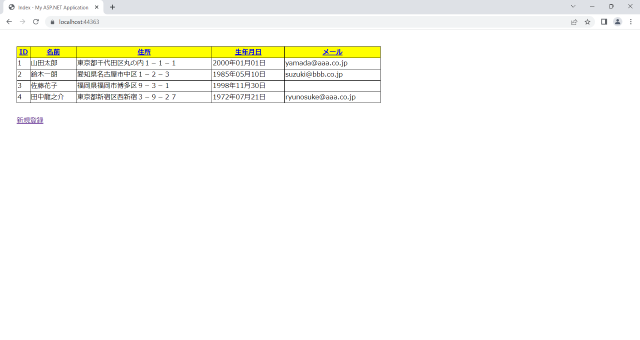