テーブルのヘッダをクリックしてソートするプログラム
今回は、テーブルのヘッダ部分をクリックすることで、その列をキーにしてソートするプログラムを作ります。
こちらの記事で作成したプログラムに、ソート処理を追加します。
プログラムを実行すると、テーブルのヘッダにソート処理を呼び出すリンクが張られます。

テーブルのモデルにアノテーションを追加
EMPテーブルモデル(Emp.vb)の各プロパティに、アノテーションのDisplayNameを追加します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
Imports System Imports System.Collections.Generic Imports System.ComponentModel Imports System.ComponentModel.DataAnnotations Partial Public Class Emp <DisplayName("ID")> Public Property Id As Integer <DisplayName("氏名")> Public Property Name As String <DisplayName("住所")> Public Property Address As String <DisplayName("生年月日")> <DisplayFormat(DataFormatString:="{0:yyyy年MM月dd日}")> Public Property Birthday As Date <DisplayName("メール")> Public Property Email As String End Class |
データ取得メソッドにソート処理を追加
データベースからデータを取得するGetEmp()メソッドに、ソートする処理を追加します。
Select Case文で、引数で渡されたソートキーを条件に処理を分岐します。
OrderBy()メソッドを使用して、ソートします。
OrderBy()メソッドの使い方は、こちらの記事を参照してください。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
Imports System.Web.Mvc Public Class DbModel Function GetEmp(skey As String) As List(Of Emp) Dim emp As List(Of Emp) Using db As New TestDbEntities Select Case skey Case "Id" emp = db.Emp.OrderBy(Function(s) s.Id).ToList Case "Name" emp = db.Emp.OrderBy(Function(s) s.Name).ToList Case "Address" emp = db.Emp.OrderBy(Function(s) s.Address).ToList Case "Birthday" emp = db.Emp.OrderBy(Function(s) s.Birthday).ToList Case "Email" emp = db.Emp.OrderBy(Function(s) s.Email).ToList Case Else emp = db.Emp.OrderBy(Function(s) s.Id).ToList End Select End Using Return emp End Function End Class |
コントローラーにソートを呼び出すメソッドを追加
コントローラーに、リンクを押下した時に呼び出されるSort()メソッドを追加します。
Sort()メソッドは、メソッド名と呼び出すビュー名が違うため、Return View()の引数にビュー名(Index)を指定する必要があります。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
Imports System.Data.Entity.Infrastructure Imports System.Web.Mvc Namespace Controllers Public Class HomeController Inherits Controller Function Index() As ActionResult Dim model As New DbModel Return View(model.GetEmp("Id")) End Function Function Sort(skey As String) As ActionResult Dim model As New DbModel Return View("Index", model.GetEmp(skey)) End Function End Class End Namespace |
ActionLink()メソッドを使ってリンクを作成
クリックでソート処理を呼び出すために、HTMLヘルパーのActionLink()メソッドを使います。
ActionLink()メソッドの使い方は、以下の通りです。
Html.ActionLink(リンク文字列, 呼び出すメソッド, (コントローラー名), New With { パラメーター = 値 }(, HTML属性 = 値)
コントローラー名は、ビューに対応するコントローラーであれば省略可能です。
HTML属性には、例えば「New With {.target = "_blank"}」とすると、別ウィンドウに結果が表示されます。
コントローラー名を指定した場合は、HTML属性を省略することはできません。
HTML属性が必要ない場合は、Nothingを指定します。
New Withで指定するパラメーターには、ドット(.)を付ける必要があります。
以下のプログラムでは、リンク文字列をHtml.DisplayNameFor()メソッドから取得していますが、文字列にするためToStringを指定しています。
ActionLink()メソッドの詳しい内容は、以下のマイクロソフトの公式サイトを参照してください。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
@ModelType IEnumerable(Of Emp) @Code ViewData("Title") = "Index" End Code <table> <tr> <th>@Html.ActionLink(Html.DisplayNameFor(Function(model) model.Id).ToString, "Sort", New With {.skey = "Id"})</th> <th>@Html.ActionLink(Html.DisplayNameFor(Function(model) model.Name).ToString, "Sort", New With {.skey = "Name"})</th> <th>@Html.ActionLink(Html.DisplayNameFor(Function(model) model.Address).ToString, "Sort", New With {.skey = "Address"})</th> <th>@Html.ActionLink(Html.DisplayNameFor(Function(model) model.Birthday).ToString, "Sort", New With {.skey = "Birthday"})</th> <th>@Html.ActionLink(Html.DisplayNameFor(Function(model) model.Email).ToString, "Sort", New With {.skey = "Email"})</th> </tr> @For Each item In Model @<tr> <td>@Html.DisplayFor(Function(model) item.Id)</td> <td>@Html.DisplayFor(Function(model) item.Name)</td> <td>@Html.DisplayFor(Function(model) item.Address)</td> <td>@Html.DisplayFor(Function(model) item.Birthday)</td> <td>@Html.DisplayFor(Function(model) item.Email)</td> </tr> Next </table> |
プログラムの実行結果
プログラムの実行結果は、以下の通りです。
以下の例では、ヘッダの「生年月日」をクリックすると、生年月日順でソートされています。
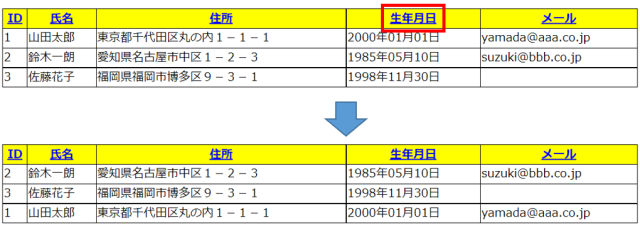